Three Steps to a Useful Minimal Feature
Recently, in the mailing list for Steve Freeman and Nat Pryce’s book Growing Object-Oriented Systems Guided by Tests, I followed a discussion that included this comment:
I guess the skill is knowing in what makes as small as possible but valid slice (as you say in the book)
Even though I’ve written before about splitting stories, I have refined my ideas about how to deliver a useful, significant, but small slice of software. I use a simple technique which I describe this way:
Write out any, and I mean any, meaningful end-to-end scenario in detail with concrete values at every step.
Now that you’ve chosen one real scenario, contract the scenario: go to each step in that scenario and ask the question, “What would I need to assume to eliminate this step?” If you find those assumptions make the scenario easier to implement, even if it makes the scenario harder to use, then use that assumption to simplify the scenario. (Don’t panic.) Repeat until exhausted. Now you have reached the kernel of the feature. Plan to build this first.
Now that you’ve found the kernel, expand it: pick one of your useful, variable, helpful variations and write a scenario that adds that variation to the kernel. Avoid building variations on top of each other, and instead favor building variations directly onto the kernel. This will not always be possible, but do your best: dependent chains of tasks create bottlenecks, so aim for scenarios that you can build independently of each other. The more you do this, the more you increase your options to deliver something sooner, thereby simplifying planning and increasing Cash In Your Pocket SoonerSM.
Based on the names of steps 2 and 3, I call this technique “Contract, then Expand”. It simultaneously helps the project community build a precise, shared understanding of the features and maximizes options for planning the work. By slicing stories or features in this way, I can use any value-hunting strategy appropriate for the situation: focus on new markets, exploit existing markets, elevate marketing risks, elevate technical risks, whatever. Once we build the kernel, we can do the rest in any sequence. Cash In My Pocket Sooner.
I’ve used the example of online bill payment in many of my classes and applied this technique. You’d be surprised how simple, but useful a bill payments system one can build.
In fact, let’s look at this example in a bit more detail.
Paying a Bill Online
What does a typical bill payments system look like? I imagine that TD Canada Trust has a fairly representative system, so I’ll use that as my example. (I originally wrote this example in 2010. The basic UI remained the same in early 2019. Banks.)
First, select the “Pay Bills” option.
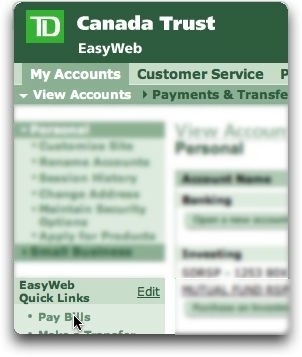
Next, select the account to use to pay the bill.
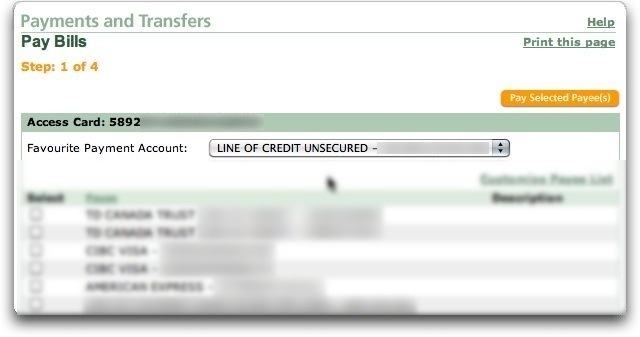
Next, select the bills you’d like to pay. Once in a while, I want to pay multiple bills at once, such as when my business has to pay the property taxes on a handful of rental properties.
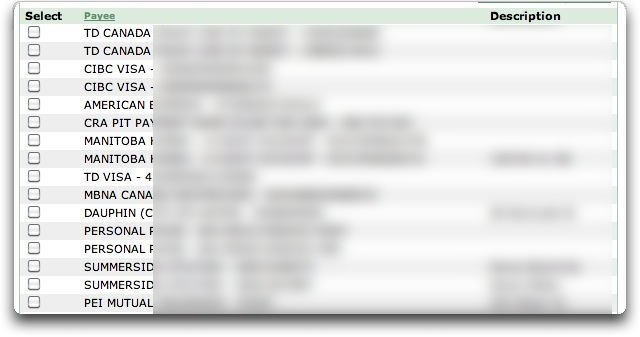
Next, enter the amount you want to pay, the date on which to pay it, the account from which to pay it (why again?) and whether you want to repeat this payment automatically.
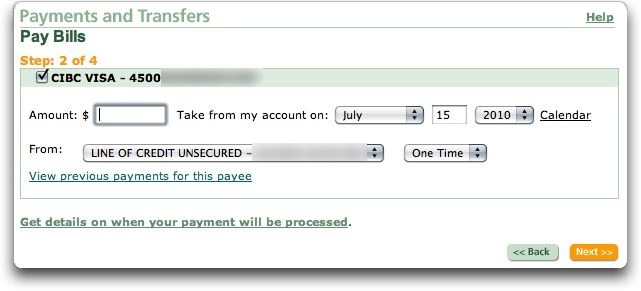
Verify the payment details: the amount, the account, the creditor, the date, and the system schedules the payment.
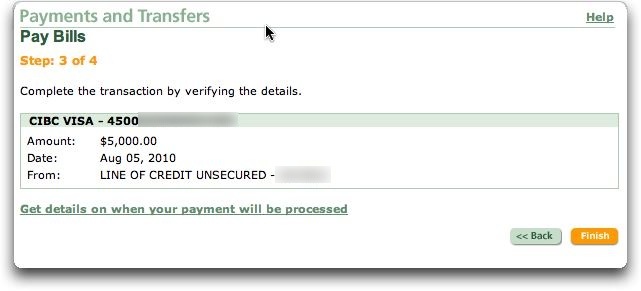
Step 1: Write a Scenario—Any Scenario
From this, I can describe the scenario in a form that looks like an executable example:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
- Joe selects chequing account 12345 from which to pay the bill
- Joe selects Visa bill with account ending in 2222 as the bill to pay
- Joe says “I want to pay $5,000”
- Joe selects a date 14 days from now as the date on which to pay the bill
- Joe selects “only once” as the frequency with which to pay the bill
- After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”
- Now the system schedules to pay the bill as requested and sends Joe an email to confirm the transaction with a link to cancel or change the scheduled payment
Apart from the numbers, this scenario perfectly accurately reflects how I pay my bills online, although I only wish TD Canada Trust would send me the confirmation email I threw in as the system’s response. We have completed step 1 of the technique: we have specified a complete scenario with concrete values at every step.
You’ll notice that we didn’t specify Joe’s username and password. We don’t intend to re-test logging in here, so we don’t bother with those details. We will have tested that elsewhere.
Step 2: Simplify the Scenario
Now we move to step 2 of the technique: looking for assumptions we could make about paying a bill online that would eliminate steps in the process. To do this, we have to be prepared to sacrifice any semblance of a decent user experience. Don’t worry: once the Walking Skeleton runs, you’ll be able to add all the bells and whistles that will make this feature a pleasure to use. For now, we want to eliminate any detail that distracts us1 from connecting our feature to the key interfaces it must deal with. In this example, I know I want to expose an HTTP interface to clients (eventually the web) and that I need to connect to the Big Ugly Banking System, but beyond that, I don’t know that anything else matters. Within this context, then, we can start making our simplifying assumptions.
That is, until someone remembers that, being a bank, we need to keep a strictly accurate record of all transactions. That means we should add a final step to the scenario: the system records the transaction in its log. While some might consider this a superfluous detail, banking regulators would call it quite essential, and so I find it hard to ignore. This means that we have a third essential interface to which to connect: the transaction logging facility. For our purposes, I’ll assume that we have one and that it has the usual properties: transaction posting date, description, and debit or credit amount, who performed the transaction and when.
This itself makes me ask whether we need to log the transaction yet, because in our scenario we’ve scheduled a payment, and not made one. Scheduling a transaction leads to issues of canceling, editing, and building a process that completes the transaction on the day the customer scheduled it. This leads to our first simplifying assumption: Joe pays the bill immediately.
This simplifies the scenario because we no longer need Joe to tell the system when to pay the bill: the system always pays the bill immediately. Our revised scenario looks like this:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
- Joe selects chequing account 12345 from which to pay the bill
- Joe selects Visa bill with account ending in 2222 as the bill to pay
- Joe says “I want to pay $5,000”
Joe selects a date 14 days from now as the date on which to pay the bill- Joe selects “only once” as the frequency with which to pay the bill
- After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”
- Now the system pays the bill as requested and sends Joe and email to let him know that the bill was paid2
I like to start at the top and look for simplifying assumptions. First, I see that Joe has to select the account from which to pay the bill, which implies that the system presents a list of accounts to Joe, which we recognize we need to do, but not in the Walking Skeleton. Ultimately, Joe simply needs to specify the account number to debit to pay the bill, so for now we’ll make him type that in. (If the user experience is starting to make you nervous, that’s fine—remember that at this point, we want to make it easier to build. We’ll make it easier to use later.) Our revised scenario looks like this:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
Joe selects chequing account 12345 from which to pay the billJoe says “I want to pay from account 12345”- Joe selects Visa bill with account ending in 2222 as the bill to pay
- Joe says “I want to pay $5,000”
- Joe selects “only once” as the frequency with which to pay the bill
- After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”
- Now the system schedules pays the bill as requested and sends Joe and email to let him know that the bill was paid
Notice that “I want to pay from account 12345” works for both user interface designs. It works if the system presents a list of accounts to choose from and it works if the system presents a text field and forces Joe to enter “12345”. Good! Simplifying the scenario has led to describing the essential interaction without unnecessary details, like how exactly the user will provide that information. We are free to change implementations of this step without changing the scenario, meaning less cost to maintain the automated test for this scenario, if we choose to write one.
Next, I see that Joe again has to select the bill to pay, which implies that the system presents a list of bills to pay. We could simplify this by requiring Joe to enter the payee identification number and the account number, even though this means saddling Joe with knowledge of payee identification numbers. In particular, the system neither has to store nor present a list of bill payees, and Joe doesn’t need to register a creditor before paying them.
Our revised scenario looks like this:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
- Joe says “I want to pay from account 12345”
Joe selects Visa bill with account ending in 2222 as the bill to pay- Joe says “I want to pay to payee number 66666”
- Joe says “I want to pay to account number 2222”
- Joe says “I want to pay $5,000”
- Joe selects “only once” as the frequency with which to pay the bill
- After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”
- Now the system schedules pays the bill as requested and sends Joe and email to let him know that the bill was paid
Next, I notice that Joe has to specify the amount to pay, and I can’t think of how to eliminate that detail without complicating matters. It does make me think about potential future features, such as “pay balance off in full” and “pay minimum payment required”, which I note down before returning to this scenario. Joe will simply have to tell us exactly how much to pay towards the bill.
Next, I notice that Joe has to confirm that he only wants a one-time payment. We can eliminate this detail by assuming that Joe can only pay the bill once. We know that customers want recurring payments, but that only distracts us from implementing the Walking Skeleton. We can eliminate this step, and our revised scenario looks like this:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
- Joe says “I want to pay from account 12345”
- Joe says “I want to pay to payee number 66666”
- Joe says “I want to pay to account number 2222”
- Joe says “I want to pay $5,000”
Joe selects “only once” as the frequency with which to pay the bill- After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”
- Now the system schedules pays the bill as requested and sends Joe and email to let him know that the bill was paid
Next, I notice that Joe has to confirm the bill payment before the system will process the payment. While this step might seem essential for security reasons, remember that we don’t necessarily have a graphical web interface for our Walking Skeleton implementation, and so we might not even have the opportunity to ask for confirmation of the bill payment. On this basis, we eliminate this step by assuming that Joe has looked over the details before pressing the button to pay the bill. Our revised scenario looks like this:
Pay a bill online
- Given that Joe has already logged in
- Joe says “I want to pay a bill”
- Joe says “I want to pay from account 12345”
- Joe says “I want to pay to payee number 66666”
- Joe says “I want to pay to account number 2222”
- Joe says “I want to pay $5,000”
After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”- Now the system schedules pays the bill as requested and sends Joe and email to let him know that the bill was paid
I can’t see any further simplifications, so I choose to stop here. I suspect this constitutes a close-to-minimal protocol for the “pay a bill online” feature. The programmer in me sees this as a single message (see “Injecting Dependencies, Partially Applying Functions, and It Really Doesn’t Matter” for how this makes it easier to test and design a solution for it), which pleases me, because of the simplicity of the interaction. The customer in me can see clearly all the extra stories we need to complete to make this feature available for public use, which makes planning easier. (I’ve even written those down as I went along.) It feels like a win for everyone, except perhaps for Joe, who has a crappy interface to work with.
Look At All The Great Ideas!
Now that we have a Walking Skeleton bill payment feature, we can identify the stories we want to deliver beyond the simplest case, and can decide which ones we need to roll this feature out to paying customers.
- Let Joe choose from a list of available payees which company to pay
- Remember the payees that Joe has previously paid and present them as “favorites” so he doesn’t have to search for them as often
- Remember the payee accounts that Joe has previously paid so that he doesn’t have to enter them each time
- Let Joe delete accounts he no longer needs to pay
- Present Joe a list of accounts he hasn’t paid in a year (longer?) and suggest deleting them
- Give Joe the option of paying the minimum payment required by the payee, whatever that happens to be
- Give Joe the option of paying the full balance owing, whatever that happens to be
- Let Joe schedule his payment in the future (by choosing a date or with presets like “tomorrow”, “next week” or using natural language like “in 12 days”)
- Let Joe schedule his payment in the future by saying “just in time”, and let the system figure out the latest date it’s safe to schedule the payment
- Let Joe cancel pending payments
- Let Joe change pending payments
- Send a reminder to Joe to pay a bill he has paid at least three of the past six months (try to detect a recurring payment)
- Let Joe schedule a payment to recur every month (same day each month)
- Notify Joe in advance of automatically detected recurring payments and ask him if he wants us to pay the bill for him
- When emailing Joe about a bill payment, include links to review the scheduled payment, change it, or cancel it, if appropriate
I imagine we could come up with more together, but I find one common thread with all these stories: once we implement the Walking Skeleton, we can implement most of these stories independently of the others. We know that having more independent stories means having greater opportunities to change priorities as needed, as well as greater opportunities to drop features in favor of other more lucrative options. Once again, everyone wins.
What Did We Do?
I like to call this technique “Contract, then Expand”, because we do these things:
- Write a scenario.
- Contract the scenario until we reach the kernel.
- Expand the scenario by adding back valuable variations in any sequence we like.
We didn’t expand here, but rather described a bunch of potential “expansions”. They form an inbox for future sessions exploring the feature. We can write scenarios for them all… later.
Need Help?
If you’re not using techniques like these, then you’re missing out on most of the value of agile software development. Really! One CEO told me that learning techniques like these saved his company multiples of $100,000 in investment in software development. This is no joke. (If you don’t care about a few hundred thousand dollars either way, that’s fine, but it’s still a lot of money to me.)
Don’t waste your agile transition building the wrong product better. Learn more about Value-Driven Product Development training.
If you’re not ready for training, but you’d like me as your trusted adviser, get fractional consulting, coaching, and mentoring. It costs less than you’d think!One More Little Thing…
I don’t like “Given that Joe has already logged in”. This refers to an implementation detail that adds nothing to the scenario and, on the contrary, distracts us from the task at hand.
Pay a bill online
Given that Joe has already logged in- Given that Joe is authorized to use bank account number 12345 to pay a bill
- Joe says “I want to pay a bill”
- Joe says “I want to pay from account 12345”
- Joe says “I want to pay to payee number 66666”
- Joe says “I want to pay to account number 2222”
- Joe says “I want to pay $5,000”
After Joe sees a summary of the bill payment he has asked to schedule, he says “I confirm that I want to pay this bill”- Now the system schedules pays the bill as requested and sends Joe and email to let him know that the bill was paid
Imagine giving other people authorization to pay bills on your behalf. (Power of attorney?) Or just to pay certain bills on your behalf. Imagine not needing to give that person the keys to your entire online banking presence. Wouldn’t that be nice? Nothing in this scenario requires nor prevents it. Nice, no?
Moreover, this makes me think about the case of not having enough money in the account when it comes time to pay the bill. (Fine… two things.) Since this scenario merely schedules the bill payment, rather than actually paying it, handling the “insufficient funds” case belongs somewhere else. Even so, it might be nice to add a warning, “You don’t have enough funds in the account to pay this bill. Please ensure that you have $5,000 in the account by December 18, 2015 in order to avoid extra charges for insufficient funds, as well as any interest and penalties that your payee might charge you.” Wouldn’t that be nice? How about an email reminder three days before the bill payment date, but only if there doesn’t appear to be an incoming transfer that would cover the amount…. Please don’t make me start a bank.
What should we do with those distractions? I write them down to get them out of my head and get back to the task at hand. I don’t want to forget to do it. It might end up on a backlog somewhere.↩︎
The system probably doesn’t pay the bill immediately, but rather schedules the bill to be paid at the earliest opportunity, and Joe cannot cancel this transaction. As far as Joe is concerned, the bank has promised to pay the bill on his behalf. On a real project, I would make this distinction, but in this example, it only serves to distract, so I have simplified even further.↩︎